The seq_along() function in R is used to generate a sequence of integers along the length of its argument.
Syntax
seq_along(len)
Parameters
len: It takes a length as an argument.
Return value
It returns an integer vector unless it is a long vector when it will be double.
Example 1: Basic usage of seq_along()
seq_along(LETTERS[1:5])
Output
[1] 1 2 3 4 5
In this example, we are creating vectors using LETTERS.
You can see that it returns the indices of the vector elements.
Example 2: Using with c()
seq_along(c(1.1, 2.1, 1.9, 1.8))
Output
[1] 1 2 3 4
Example 3: Looping
vec <- c(1.1, 2.1, 1.9, 1.8)
# Looping over a vector using seq_along
for (i in seq_along(vec)) {
print(paste("Element", i, "is", vec[i]))
}
Output
[1] "Element 1 is 1.1"
[1] "Element 2 is 2.1"
[1] "Element 3 is 1.9"
[1] "Element 4 is 1.8"
Example 4: Handles Zero Length
If the input vector is of length 0, this function returns an integer vector of length zero, often the desired behavior in loops or other constructs where the input might be empty.
# Empty vector
empty_vec <- numeric(0)
# Sequence along an empty vector
sequence_empty <- seq_along(empty_vec)
# Print the sequence (will be an empty integer vector)
print(sequence_empty)
Output
integer(0)
Using seq_along() is safer than using 1:length(x) because if x is of length zero, 1:length(x) returns 1:0, which is a sequence of two elements (1 and 0), not an empty sequence.
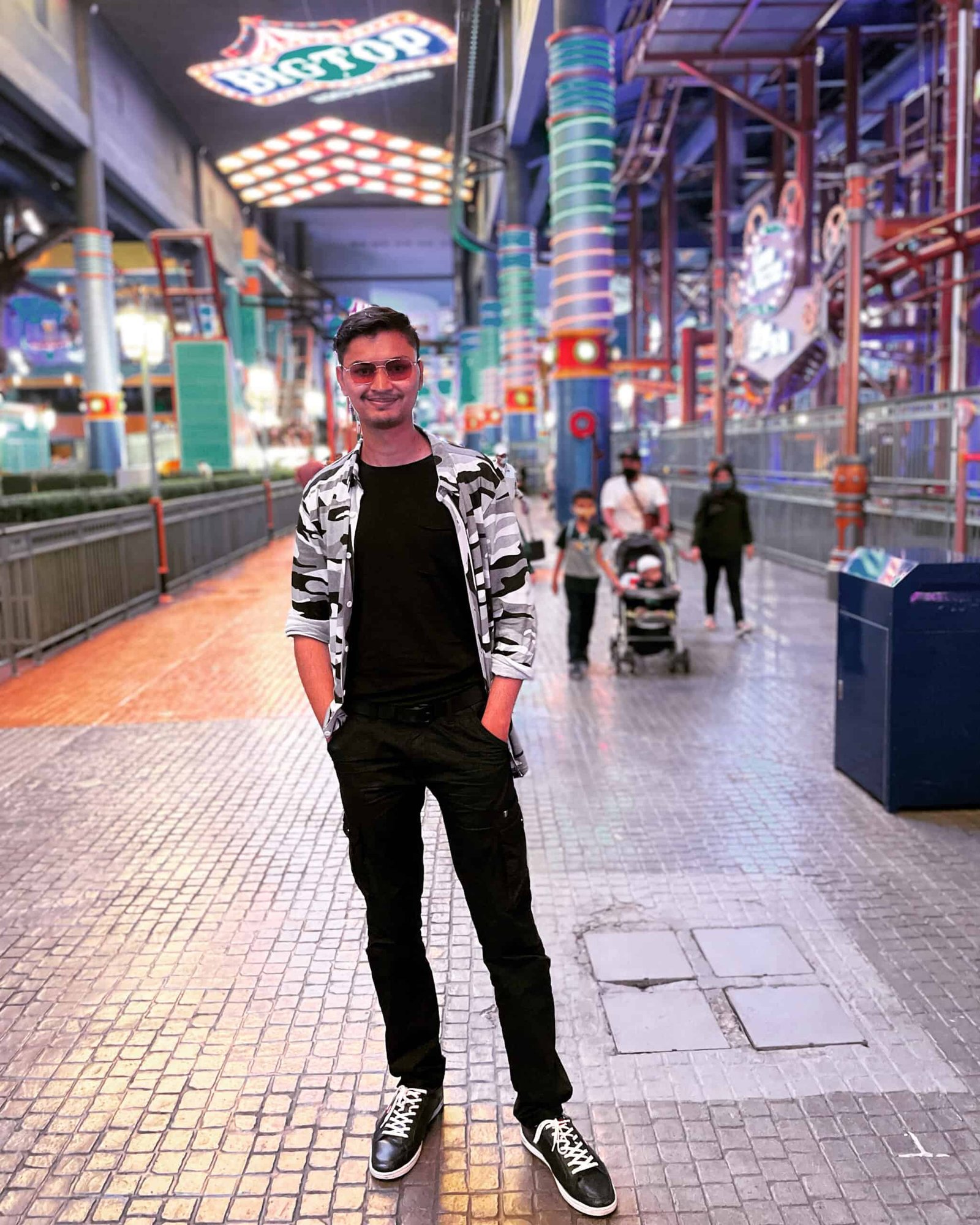
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.