Dates in R are stored as the number of days since 1970-01-01, so converting a Date object to a Numeric gives those day counts. But what about POSIXct? Well, those are stored as seconds since the same epoch, so converting them would give a much larger number.
Here are three ways to convert Date to Numeric:
- Using as.numeric()
- Using lubridate package
- Using Julian dates with julian()
Method 1: Using as.numeric()
The as.numeric() function accepts date objects that can be created using the as.Date() or as.POSIXct() function and converts it into a numeric value.
Date Objects
If you want to get days from 1970-01-01, create a date object using the as.date() function and pass it to the as.numeric() function. This will return the total number of days since 1970-01-01.
date <- as.Date("2025-03-10")
numeric_date <- as.numeric(date)
print(numeric_date)
# Output: [1] 20157 (days since 1970-01-01)
POSIXct Objects
The POSIXct represents seconds since the same epoch. To create a POSIXct object, we need a date and timezone arguments.
datetime <- as.POSIXct("2025-03-10", tz = "UTC")
numeric_datetime <- as.numeric(datetime)
print(numeric_datetime)
# Output: [1] 1741564800 (seconds since epoch)
Applying an as.numeric() function to a POSIXct object will return the number of seconds since January 1, 1970 (the Unix epoch) at the specified date and time.
However, you need to know the time zone used in your POSIXct object to get the correct result.
To get the number of days from the number, divide the number by 86400:
days <- numeric_datetime / 86400
print(days)
# Output: [1] 20157 (Number of days since epoch)
To get the number of years from the date, divide it by 365:
years <- numeric_datetime / 86400 / 365 print(years) # Output: [1] 55.22466
Combine Date Components (YYYYMMDD)
Let’s extract the year, month, and day from today’s date and combine them into a numeric value using the as.numeric() function.
date <- as.Date("2025-03-10")
yyyymmdd <- as.numeric(format(date, "%Y%m%d"))
print(yyyymmdd)
# Output: [1] 20250310
Here, you can use the sprintf() function for custom padding if needed.
Extract Date Components
You can break dates into numeric vectors for year, month, day, etc., using as.numeric() and format() functions.
date <- as.Date("2025-01-01")
year <- as.numeric(format(date, "%Y"))
print(year) # Output: 2025
month <- as.numeric(format(date, "%m"))
print(month) # Output: 1
day <- as.numeric(format(date, "%d"))
print(day) # Output: 1
Handling Factors and Character Strings
Converting factors directly to numeric returns levels, not dates. If your input is in the format of a factor, your first step should be to convert the factor into a proper date object and then use the as.numeric() function to get its numerical representation.
date_factor <- factor("2025-03-10")
date <- as.Date(as.character(date_factor))
numeric_date <- as.numeric(date)
print(numeric_date) # [1] 20157
In real life, people might want to represent dates as factors and then convert those factors to numeric. But that’s different.
For example, if you have a factor of dates, converting directly to numeric would give the factor levels, not the actual dates. So that’s a pitfall to avoid. The user should first convert the factor to Date, then to numeric.
Time Zone Considerations for POSIXct
The numeric conversion of POSIXct uses UTC by default. Specify time zones explicitly:
datetime <- as.POSIXct("2020-01-01 00:00:00", tz = "America/New_York")
numeric_datetime <- as.numeric(datetime)
print(numeric_datetime)
# Output: 1577854800
Date Differences as Numeric
You can calculate the interval of days between two days using the difftime() method with the help of the as.numeric().
date1 <- as.Date("2025-03-01")
date2 <- as.Date("2025-03-07")
diff_days <- as.numeric(difftime(date2, date1, units = "days"))
print(diff_days)
# Output: 6
Method 2: Using a lubridate package
To use the lubridate package, you must install and load the lubridate package:
install.packages("lubridate")
library(lubridate)
Syntax
library(lubridate)
#get year value in date object
year(date)
#get seconds value in date object
second(date)
#get minutes value in date object
minute(date)
Code example
library(lubridate)
today_date <- as.POSIXct("2025-03-10 13:09:00", tz = "GMT")
hour(today_date)
# Output: 13
minute(today_date)
# Output: 9
second(today_date)
# Output: 0
day(today_date)
# Output: 10
month(today_date)
# Output: 03
year(today_date)
# Output: 2025
Method 3: Using julian() function
The julian() function returns the Julian date, which is the number of days since the origin (by default 1970-01-01), which is the same as as.numeric(), but you can specify a different origin.
# Define a date for which you want to calculate Julian days
date <- as.Date("2025-03-31") # Example date - change this to your desired date
julian_days <- julian(date, origin = as.Date("2025-03-10"))
print(julian_days)
# Output: [1] 21
# attr(,"origin")
# [1] "2025-03-10"
In the above code, we defined the custom origin, 2025-03-10, and calculated the total number of days from this origin to the final date, 31 March 2025. The days count from origin to final day, which is 21 in our code example.
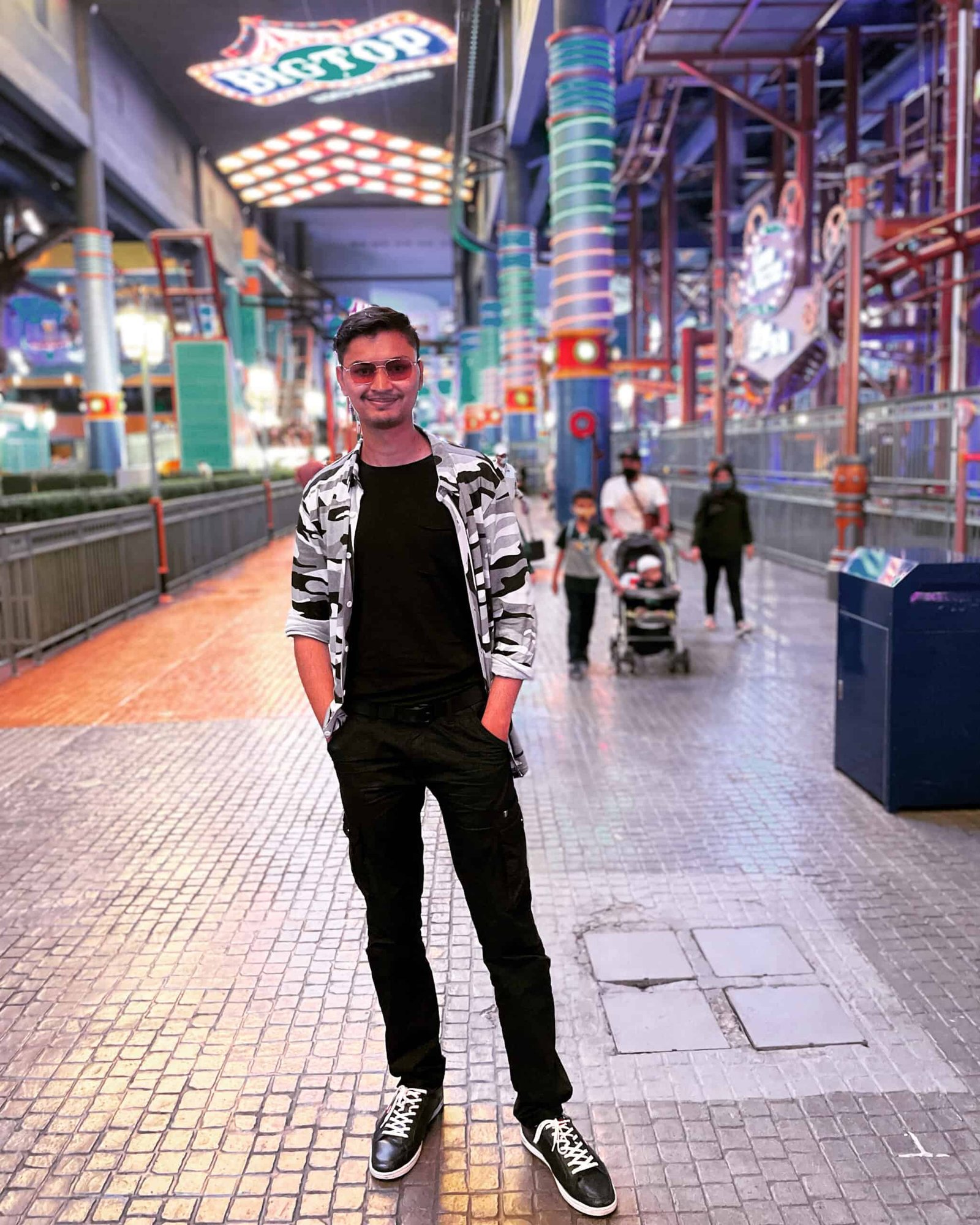
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.