R vectors are atomic, meaning they contain homogeneous data types. They are contiguous in memory.
Here are some of the best approaches for vector initialization and appending values in the future:
Using type-specific functions
The most efficient and quickest way to create an empty vector is to use type-specific functions like numeric(0), which will return a numeric empty vector.
The character(0) returns an empty character vector, and the logical(0) returns an empty logical vector.
# Numeric vector
vec_num <- numeric(0)
print(vec_num)
# Output: numeric(0)
print(class(vec_num))
# Output: [1] "numeric"
# Character vector
vec_char <- character(0)
print(vec_char)
# Output: character(0)
print(class(vec_char))
# Output: [1] "character"
# Logical vector
vec_logical <- logical(0)
print(vec_logical)
# Output: logical(0)
print(class(vec_logical))
# Output: [1] "logical"
Here is a guide for checking if a vector is empty efficiently.
Using vector() function
Another way is to use the vector() function and specify the mode and length. The mode is a data type; the length should be zero, as it is empty.
For example, a vector(“numeric”, 0) creates an empty vector of type numeric.
# Numeric vector
vec_num <- vector(mode = "numeric", length = 0)
print(vec_num)
# Output: numeric(0)
print(class(vec_num))
# Output: [1] "numeric"
# Character vector
vec_char <- vector(mode = "character", length = 0)
print(vec_char)
# Output: character(0)
print(class(vec_char))
# Output: [1] "character"
In the above code, we created two types of empty vectors:
- numeric
- character
Using the class() function, we checked the data type, and it returned the expected result.
Using c() function (Not recommended)
The c() function is not an appropriate option because c() without arguments creates a NULL object, which is also not good for type safety.
empty_vec <- c()
print(empty_vec)
# Output: NULL
Since the type is NULL, it may cause unintended coercion later. Avoid repeatedly using c() in loops.
Appending values to the vector
When it comes to appending values to an empty vector, you can either use the c() function or the append() function. Yes, the c() function is efficient for appending and concatenating values.
Using c()
# Numeric vector
vec_num <- vector(mode = "numeric", length = 0)
print(vec_num)
# Output: numeric(0)
print(class(vec_num))
# Output: [1] "numeric"
# Appending values using c() function
vec_num <- c(vec_num, 1, 2, 3, 4, 5)
print(vec_num)
# Output: [1] 1 2 3 4 5
The above code shows that we appended 1, 2, 3, 4, and 5 elements and finally printed them.
Appending Multiple Values
You can combine multiple vectors or use the c() method with multiple elements.
# Numeric vector
vec_num <- vector(mode = "numeric", length = 0)
vec1 <- c(1, 2)
vec2 <- c(3, 4)
# Combining vector using c() function
vec_num <- c(vec_num, vec1, vec2)
print(vec_num)
# Output: [1] 1 2 3 4
Using append()
With the help of the append() function, you can append values at specific positions.
# Numeric vector
vec_num <- numeric(0)
print(vec_num)
# Output: numeric(0)
print(class(vec_num))
# Output: [1] "numeric"
# Appending values using append() function
vec_num <- append(vec_num, 1:4)
print(vec_num)
# Output: [1] 1 2 3 4
# Appending values at specific position
vec_num <- append(vec_num, c(5), after = 2)
print(vec_num)
# Output: [1] 1 2 5 3 4
In the above code, we initialized an empty numeric vector and added four values using the append() function.
Then, again, we added element “5” after position 2 at a specific position. Finally, we printed the vector.
Efficient: Pre-Allocate Memory
It is always an efficient approach to pre-allocate memory using type-specific functions and then assign values to that vector.
If you know the final size of a vector in advance, you should start pre-allocating it.
After pre-allocating, use index-based assignment (vec[i] <- value) within loops to fill in the values. This directly writes to the pre-allocated memory without reallocation.
len <- 1e4
vec <- numeric(len) # Pre-allocate
for (i in 1:len) {
vec[i] <- i # Direct assignment by index
}
print(vec)
# Output: [1] 1e+04
For large vectors, you shall always pre-allocate the memory and avoid appending in loops because it will cost you performance. Instead, use lists or vectorized operations.
If the final size is unknown, use a list (flexible) and convert it to a vector later:
temp_list <- list()
for (i in 1:1e4) {
temp_list[[i]] <- i
}
vec <- unlist(temp_list) # Converts list to vector
print(vec)
# Output: [1] 1e+04
That’s all!
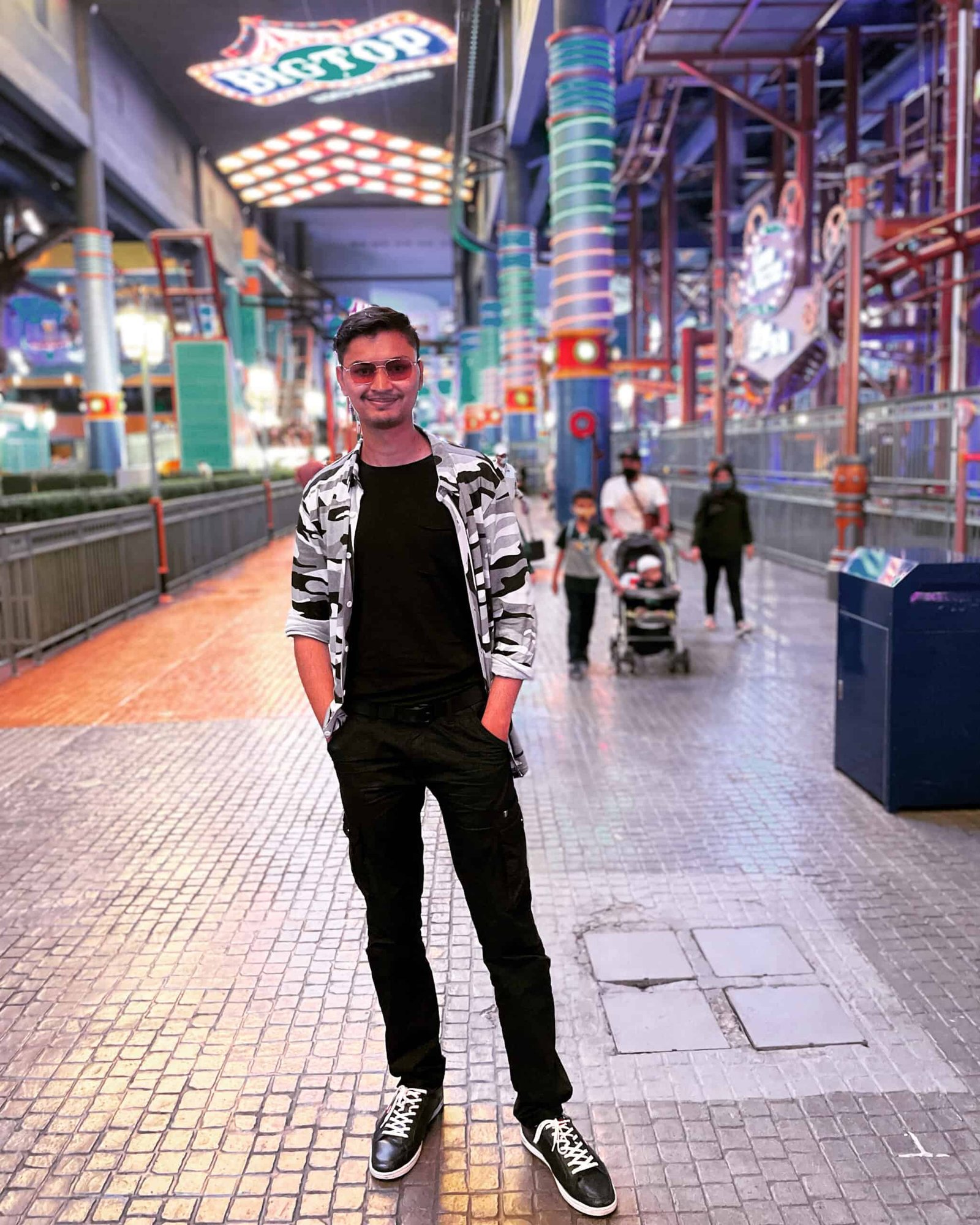
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.
A buddy of mine recommended your blog on Facebook. I can see why. Great post here.