The append() function in R concatenates values to a vector or list at a specified position. It returns a new object without modifying the original.
You can add a single or multiple elements to a vector or list.
Syntax
append(obj, values, after = length(x))
Parameters
Name | Value |
obj | It is an original vector or list. |
value | It is the value(s) to be appended to the vector or list. |
index | It is a position to insert values (default: end). |
Appending a single element to a Vector
By default, the append() function will append an element at the end of the vector if you don’t specify the position.
data <- 1:3
element <- 19
cat(data, "\n")
modified_vec <- append(data, element)
cat("After appending an element to a vector", "\n")
cat(modified_vec)
Output
1 2 3
After appending an element to a vector
1 2 3 19
Appending multiple elements to a Vector
When you append multiple elements to a vector, you can append them as a separate vector rather than as individual elements. What I mean by this is that If you have multiple elements, then create a vector of it and then append it to an existing vector. That way, you have an output vector that contains multiple appended elements.
v1 <- 1:3
v2 <- 19:21
cat("Before appending elements to v1", "\n")
cat(v1, "\n")
modified_data <- append(v1, v2)
cat("After appending multiple elements to a v1", "\n")
cat(modified_data)
Output
Before appending elements to v1
1 2 3
After appending multiple elements to a v1
1 2 3 19 20 21
Adding to the specific position
The function provides an “after” argument that you can use to pinpoint a specific position and append to it.
v1 <- 1:4
v2 <- 19
cat("Before adding element to v1", "\n")
cat(v1, "\n")
cat("Appending element after index 2", "\n")
modified_data <- append(v1, v2, 2)
cat(modified_data)
Output
Before adding element to v1
1 2 3 4
Appending element after index 2
1 2 19 3 4
Appending a single element to a List
As we discussed earlier, the append() function can also be used to add elements to a list. Its usage is not limited to a vector.
main_list <- list(a = 1, b = 2)
element <- list(c = 3)
append(main_list, element) # Adds 'c=3' to the end
Output
$a
[1] 1
$b
[1] 2
$c
[1] 3
Appending multiple elements to a List
When you append multiple elements to a list, you can append them as a separate list rather than as individual elements.
main_list <- list(a = 1, b = 2)
append_list <- list(c = 3, d = 4, e = 5)
append(main_list, append_list)
Output
$a
[1] 1
$b
[1] 2
$c
[1] 3
$d
[1] 4
$e
[1] 5
Appending elements to a specific position
Same as a vector, you can specify the “after” argument to define the exact position of insertion. It will append after that index that you defined.
main_list <- list(a = 1, b = 2, c=3)
append_list <- list(d = 4)
append(main_list, append_list, after = 1) # Inserts after the first element
Output
$a
[1] 1
$d
[1] 4
$b
[1] 2
$c
[1] 3
You can see that we appended after index 1, which is position number 2. List index starts with 1.
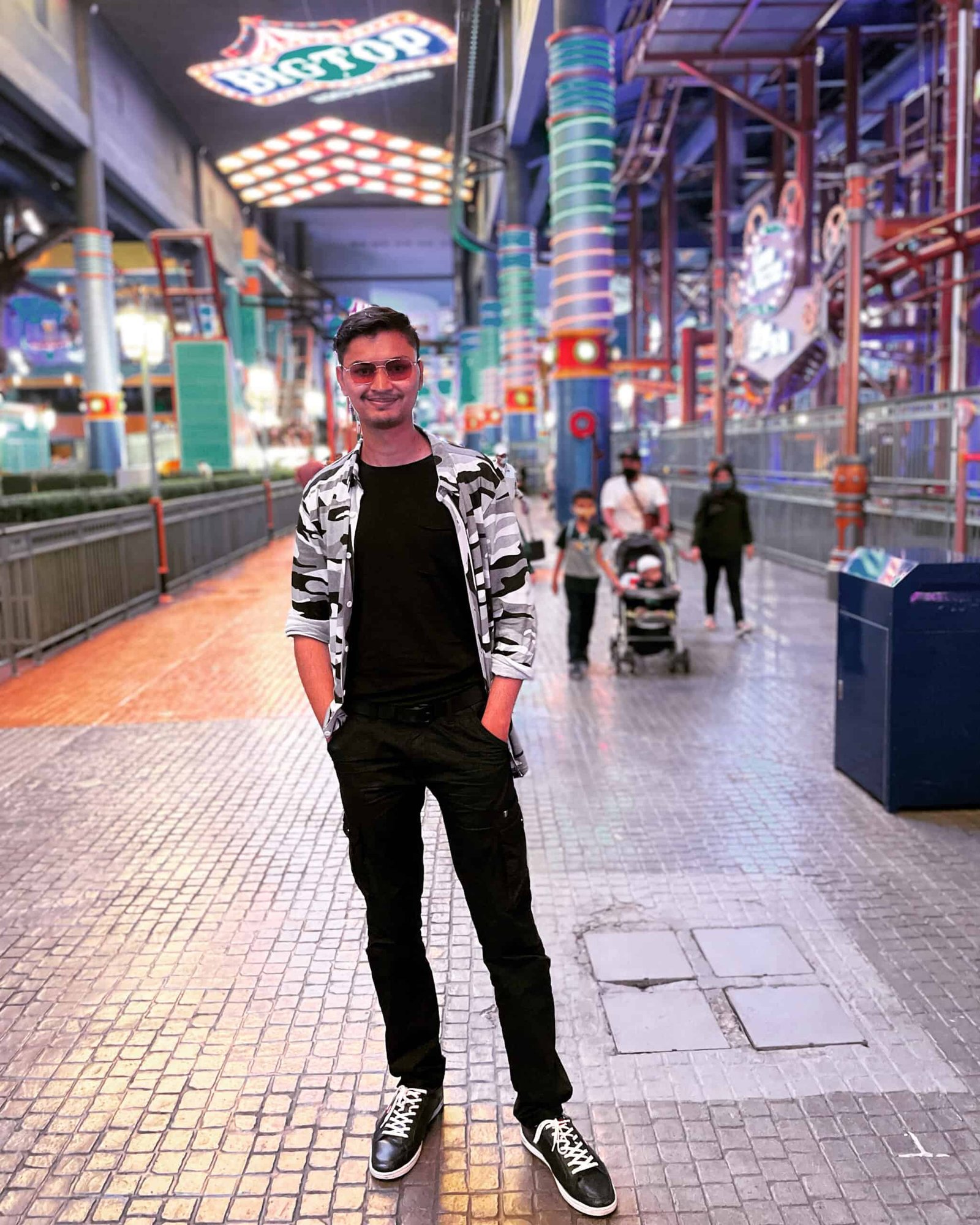
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.