R cbind (column bind) is a function that combines specified vectors, matrices, or data frames by columns, creating a new matrix or data frame where the input objects are placed side by side.
Syntax
cbind(..., deparse.level = 1)
Parameters
Argument | Description |
… | It represents the objects that we need to combine column-wise. It can be anything from vectors, matrices, to data frames. |
deparse.level | It governs how column names are generated from the input. |
Combining two data frames
Let’s merge two data frames by columns.
df1 <- data.frame(col1 = c(1, 2, 3), col2 = c(11, 19, 21))
df2 <- data.frame(col3 = c(10, 18, 46), col4 = c(5, 15, 25))
# Appending df2 to df1 using cbind() function
df_new <- cbind(df1, df2)
# View the updated data frame
print(df_new)
Combining a vector with a data frame column-wise
Let’s combine a vector and a data frame. The vector will be appended as a new column of the data frame. So, if your input data frame has two columns, the output data frame would have three columns.
df1 <- data.frame(col1 = c(1, 2, 3), col2 = c(11, 19, 21))
col3 <- c(10, 18, 46)
# Append the vectors as new rows to the data frame
df_new <- cbind(df1, col3)
# View the updated data frame
print(df_new)
Combine multiple columns
We can append two vectors to an existing data frame. Both vectors will be appended as two new columns of the data frame.
df <- data.frame(c1 = c(1, 2, 3, 4),
c2 = c(5, 6, 7, 8),
c3 = c(9, 10, 11, 12))
c4 <- c(18, 19, 20, 21)
c5 <- c(29, 46, 47, 37)
cat("After adding multiple columns using cbind()", "\n")
newDf <- cbind(df, c4, c5)
print(newDf)
Combine vectors into a matrix
We can also combine multiple vectors into a data frame using the cbind() method.
a <- c(1, 2, 3)
b <- c(4, 5, 6)
mat <- cbind(a, b)
d <- c(7, 8, 9)
matrx <- cbind(mat, d)
print(matrx)
Output
a b d
[1,] 1 4 7
[2,] 2 5 8
[3,] 3 6 9
Handling unequal lengths
We can combine a vector with a single value or a shorter vector.
x <- c(1, 2, 3)
y <- 10
combined_vec <- cbind(x, y)
print(combined_vec)
# Output:
# x y
# 1 10
# 2 10
# 3 10
You can see from the above code that the single value y = 10 is recycled to match the length of x, creating a matrix with two columns.
Using deparse.level for column naming
Let’s control how column names are assigned.
x <- c(1, 2, 3)
y <- c(4, 5, 6)
# Default: deparse.level = 1
result1 <- cbind(x, y)
print(colnames(result1))
# Output: [1] "x" "y"
# No names: deparse.level = 0
result2 <- cbind(x, y, deparse.level = 0)
print(colnames(result2))
# Output: NULL
With deparse.level = 1, column names are derived from the variable names (x, y). With deparse.level = 0; No names are assigned.
That’s it!
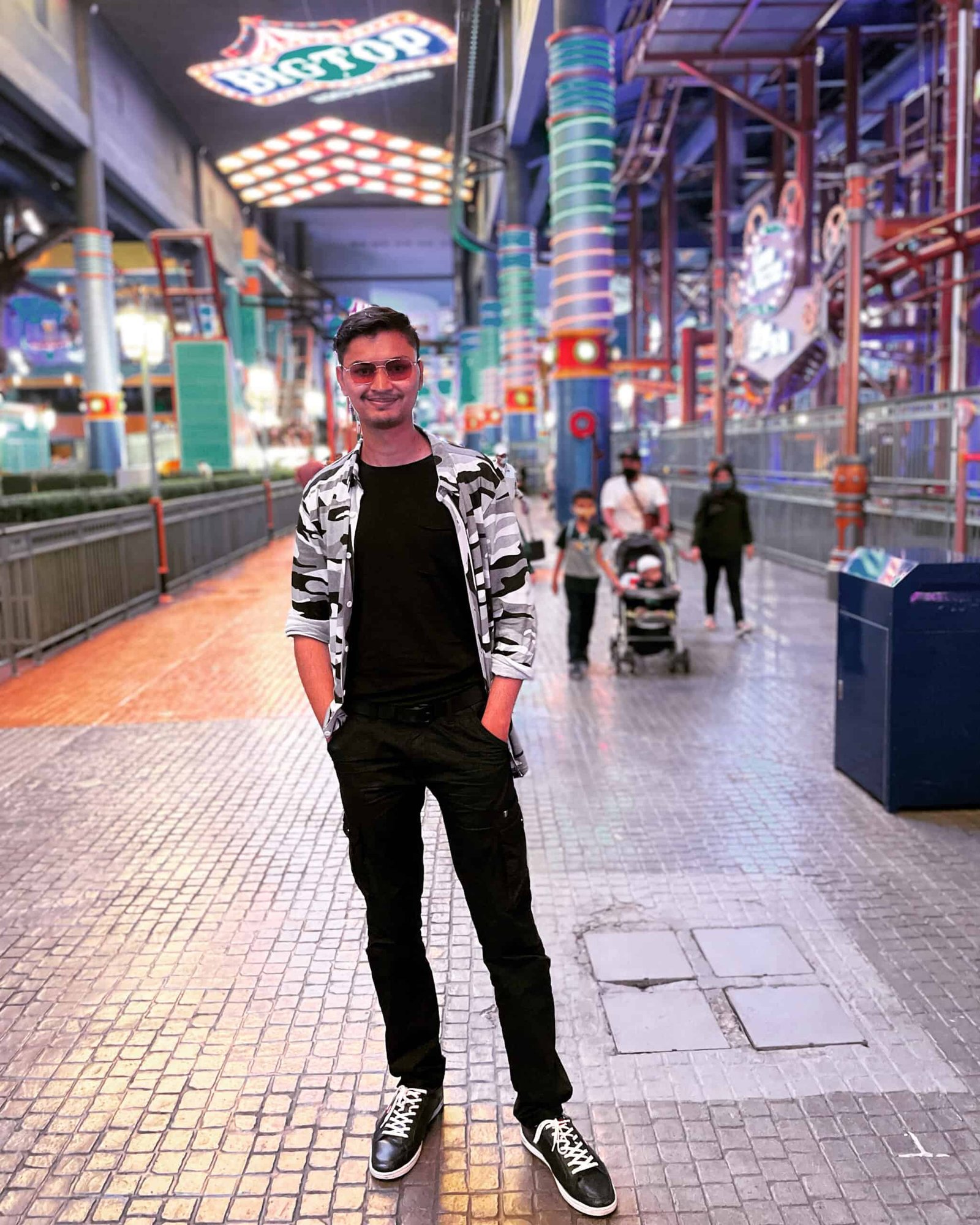
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.