The square root of a number is a value that is multiplied by itself, giving the original number. For example, the square root of 16 is 4 because if you multiply 4 by 4, it will give us 16, so the original number is 4.
To calculate the square root of a number, vector, matrix, or list in R, use the sqrt() function. The sqrt() is a base function that accepts an object and returns its square root. For example, sqrt(16) returns 4, and sqrt(c(1, 4, 9)) returns c(1, 2, 3).
Visual Representation
Syntax
sqrt(obj)
Parameters
Name | Value |
obj | It’s a numerical value greater than 0. It can be a vector, matrix, or data frame column. |
Positive number
num <- 16
cat("The square root of 16 is: ", sqrt(num))
Output
The square root of 16 is: 4
Negative number
If you pass a negative number to the sqrt() function, it will return a warning: NaNs produced. In mathematics, if you try to find a square root of a negative number, it won’t return a real number. For that, R returns NaN.
num <- -16
sqrt(num)
Output
[1] NaN
Warning message:
In sqrt(num) : NaNs produced
To fix this warning, we can use the abs() function. The abs() function returns the absolute value of an input number.
num <- -16
sqrt(abs(num))
Output
[1] 4
Complex number
If you are dealing with a complex number and it is represented correctly as a complex number, the sqrt() function will accept that number and return the square root.
z <- 3 + 4i
sqrt_root_z <- sqrt(z)
print(sqrt_root_z)
Output
[1] 2+1i
Vector
R is vectorized, which means if you pass a vector to the sqrt() function, it will return the square root of each element of the vector. It performs element-wise operations smoothly. It preserves the original structure.
rv <- c(11, 19, 21, 16, 49, 46)
sqrt(rv)
Output
[1] 3.316625 4.358899 4.582576 4.000000 7.000000 6.782330
Matrix
If you pass the whole matrix to the sqrt() function, it will return a matrix of the same size, with each element being the square root of the corresponding input matrix.
vec <- c(1, 4, 9, 16, 25, 36, 49, 64, 81)
mtrx <- matrix(vec, nrow = 3, ncol = 3)
print(mtrx)
cat("After calculating square root of matrx", "\n")
sqrt(mtrx)
Output
List
We can not find the square root of the List, and if we try to find it, it will give us the following error: non-numeric argument to mathematical function
litt <- list(11, 19, 21, 16, 49, 46)
print(litt)
cat("After calculating square root of list", "\n")
list_sqrt <- sqrt(litt)
print(list_sqrt)
Output
After calculating square root of list
Error in sqrt(litt) : non-numeric argument to mathematical function
Execution halted
Factor
We can get the error with Factor as well. But that error is different than the above.
fact <- factor(10)
sqrt(fact)
Output
Error in Math.factor(fact) : ‘sqrt’ not meaningful for factors
Execution halted
To calculate the square root of the factor and fix the error, use “as.numeric()” and “as.character()” with the sqrt() method.
fact <- factor(10)
print("The factor is: ")
print(fact)
sqrt_fact <- sqrt(as.numeric(as.character(fact)))
print("The square root of factor is: ", sqrt_fact)
print(sqrt_fact)
Output
[1] "The factor is: "
[1] 10
Levels: 10
[1] "The square root of factor is: "
[1] 3.162278
Error in sqrt(x): non-numeric argument to mathematical function
The error message “Error in sqrt(x): non-numeric argument to mathematical function” occurs when the sqrt() function has been called with an argument that is not a numeric value.
some_var <- "16"
sqrt(some_var)
Output
Error in sqrt(some_var) : non-numeric argument to mathematical function
To fix this error, convert the string to an integer before calculating the square root using the as.numeric() function.
some_var <- "16"
sqrt(as.numeric(some_var))
Output
[1] 4
Handling missing and NaN values
Missing values are denoted by NA, and If I have an NA value and try to find its square root, it returns NA.
num <- NA
sqrt(num)
Output
[1] NA
If you pass NaN, it will return NaN.
num <- NaN
sqrt(num)
Output
[1] NaN
Data Frame
The data frame consists of rows and columns, and each column can have different data types. If you pass the whole data frame to the sqrt() function and some of the columns of that data frame have non-numeric types, it will return an error.
df <- data.frame(
name = c("Krunal", "Ankit", "Rushabh", "Dhaval", "Tejas", "Rushabh", "Dhaval", "Tejas"),
score = c(85, 90, 78, 92, 88, 78, 92, 88),
subject = c("Math", "Math", "History", "History", "Math", "History", "History", "Math"),
grade = c("10th", "11th", "11th", "10th", "10th", "11th", "10th", "10th")
)
print(sqrt(df))
Output
Error in Math.data.frame(df) :
non-numeric-alike variable(s) in data frame: name, subject, grade
Calls: print -> Math.data.frame
Execution halted
The proper solution to this problem is either to apply sqrt() to specific columns that are numeric or convert the data frame appropriately before executing that function.
df[, numeric_cols] <- sqrt(df[, numeric_cols])
Conclusion
The sqrt() is optimized via C code (.Primitive(“sqrt”)), making it faster than x^0.5 or x^(1/2) for large datasets. It is a base R function, so you don’t have to import via third-party packages.
The statistical applications of this function are finding standard deviation or Euclidean distance in clustering algorithms.
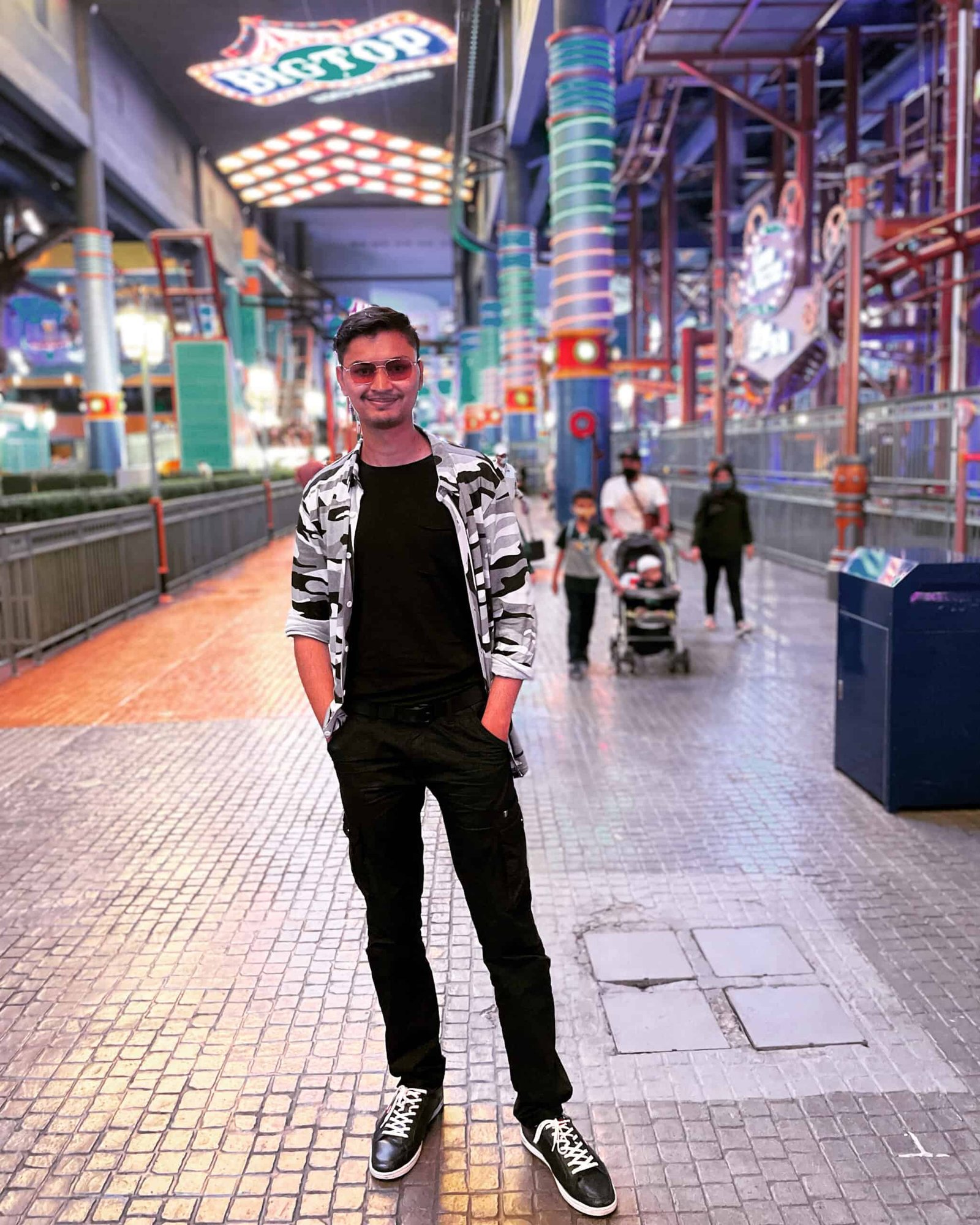
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.