For data exploration, quickly inspecting the data, verifying the structure, or debugging large datasets, we need some kind of tool that helps prevent excessive data flooding in the console. That’s where head() and tail() generic functions come into play.
head() function
The head() function displays the first n parts of the vector, matrix, table, or data frame. If you don’t pass the count “n” or rows to be displayed, it will display six rows/elements by default.
Syntax
head(data, n = number, ...)
Parameters
Name | Value |
data | An input object (data frame, vector, matrix, or list) |
n | Number of rows/elements to be returned.
|
… | They are additional arguments. For example, if you use matrices, you can use nrow or ncol. |
Return value
- For vectors, head() returns a subset of the first n elements.
- For data frames/matrices, it returns a subset of the first n rows.
- For lists, it returns the first n elements of the list.
If the “n” argument exceeds the input object’s length, it will return the whole object.
Basic usage with a data frame
Let’s define a data frame and use the head() function to quickly inspect the data frame.
# Creating a data frame.
df <- data.frame(
col1 = c(1, 2, 3, 4, 5, 6, 7),
col2 = c(8, 9, 10, 11, 12, 13, 14),
col3 = c(15, 16, 17, 18, 19, 20, 21)
)
head(df)
Selecting the first n rows
# Create a data frame.
df <- data.frame(
col1 = c(1, 2, 3, 4, 5, 6, 7),
col2 = c(8, 9, 10, 11, 12, 13, 14),
col3 = c(15, 16, 17, 18, 19, 20, 21)
)
# Selecting first 3 rows
head(df, 3)
Selecting the first n values in the specific column
# Create a data frame.
df <- data.frame(
col1 = c(1, 2, 3, 4, 5, 6, 7),
col2 = c(8, 9, 10, 11, 12, 13, 14),
col3 = c(15, 16, 17, 18, 19, 20, 21)
)
# Selecting first 4 rows of column 3
head(df$col2, 4)
Negative “n” in Data Frames
If you pass the negative “n”, it will start excluding the rows from the end of the data frame. For example, if you want to exclude the last five rows from a data frame, you should pass n = -5.
# Create a data frame.
df <- data.frame(
col1 = c(1, 2, 3, 4, 5, 6, 7),
col2 = c(8, 9, 10, 11, 12, 13, 14),
col3 = c(15, 16, 17, 18, 19, 20, 21)
)
# excluding last 2 rows
head(df, -5)
It will return the following output:
col1 col2 col3
1 1 8 15
2 2 9 16
The above output shows that it removed the last five rows and displayed the first two rows.
Usage with the dataset
df <- datasets::USArrests
head(df, 5)
Output
Usage with vector
rv <- 1:5
cat("First three values of vector", "\n")
head(rv, 3)
Usage with a matrix
Let’s create a matrix of 7×3 and fetch the first four rows of the Matrix.
rv <- 1:21
mtrx <- matrix(rv, nrow = 7, ncol = 3)
cat("Using head() function to get first 4 rows", "\n")
head(mtrx, 4)
Usage with a list
Let’s define a list and quickly view the first 2 elements of the list:
# Creating a list
demo_list <- list(a = 1:3, b = 4:6, c = 7:9, d = 10:12)
demo_list
# $a
# [1] 1 2 3
# $b
# [1] 4 5 6
# $c
# [1] 7 8 9
# $d
# [1] 10 11 12
# Get first 2 elements
head(demo_list, n = 2)
# $a
# [1] 1 2 3
# $b
# [1] 4 5 6
tail() function
The tail() function views the last n part of a vector, matrix, table, or data frame.
It complements the head() function that returns the first n rows of a data frame.
Syntax
tail(data, n, ...)
Parameters
Name | Value |
data | An input object (data frame, vector, matrix, or list). |
n | Number of rows/elements to be returned.
|
… | They are additional arguments. For example, if you use matrices, you can use nrow or ncol. |
Return value
- For vectors, head() returns a subset of the last n elements.
- For data frames/matrices, it returns a subset of the last n rows.
- For lists, it returns the last n elements of the list.
If the “n” argument exceeds the input object’s length, it will return the whole object.
Viewing the last rows of the data frame
By default, it will return the last six rows of the data frame if you have not passed a number of rows/elements.
# Create a data frame.
df <- data.frame(
col1 = c(1, 2, 3, 4, 5, 6, 7),
col2 = c(8, 9, 10, 11, 12, 13, 14),
col3 = c(15, 16, 17, 18, 19, 20, 21)
)
# Applying tail() function to df
tail(df)
Selecting the last n rows from a data frame
To select the last three rows of a data frame, pass three as the second argument.
# Create a data frame.
df <- data.frame(
col1 = c(1, 2, 3, 4, 5, 6, 7),
col2 = c(8, 9, 10, 11, 12, 13, 14),
col3 = c(15, 16, 17, 18, 19, 20, 21)
)
# Selecting last 3 rows using tail() function
tail(df, 3)
Get the last n values in the specific column
Let’s implement the above diagram in R code.
# Create a data frame.
df <- data.frame(
col1 = c(1, 2, 3, 4, 5, 6, 7),
col2 = c(8, 9, 10, 11, 12, 13, 14),
col3 = c(15, 16, 17, 18, 19, 20, 21)
)
# Selecting last 4 rows of column 3
tail(df$col3, 4)
Excluding rows
If you pass the negative n, it will start excluding rows from the start. For example, tail(df, -5) will exclude the first five rows from the data frame.
# Create a data frame.
df <- data.frame(
col1 = c(1, 2, 3, 4, 5, 6, 7),
col2 = c(8, 9, 10, 11, 12, 13, 14),
col3 = c(15, 16, 17, 18, 19, 20, 21)
)
print(df)
# excluding first 5 rows
tail(df, n = -5)
Output
col1 col2 col3
6 6 13 20
7 7 14 21
As you can see, it removes the first five rows and displays the last two rows.
Usage with built-in dataset
Let’s import the ChickWeight dataset.
df <- datasets::ChickWeight
Use the tail() function to get the last five rows of the dataset.
df <- datasets::ChickWeight
tail(df, 5)
Output
weight Time Chick Diet
574 175 14 50 4
575 205 16 50 4
576 234 18 50 4
577 264 20 50 4
578 264 21 50 4
Usage with vector
rv <- 1:10
rv
cat("Last five integers of vector", "\n")
tail(rv, 5)
Output
[1] 1 2 3 4 5 6 7 8 9 10
Last five integers of vector
[1] 6 7 8 9 10
Usage with a matrix
rv <- 1:21
mtrx <- matrix(rv, nrow = 7, ncol = 3)
cat("Using tail() function to get last 3 rows", "\n")
tail(mtrx, 3)
Usage with a list
# Creating a list
demo_list <- list(a = 1:3, b = 4:6, c = 7:9, d = 10:12)
demo_list
# $a
# [1] 1 2 3
# $b
# [1] 4 5 6
# $c
# [1] 7 8 9
# $d
# [1] 10 11 12
# Get last 2 elements
tail(demo_list, n = 2)
# $c
# [1] 7 8 9
# $d
# [1] 10 11 12
That’s it!
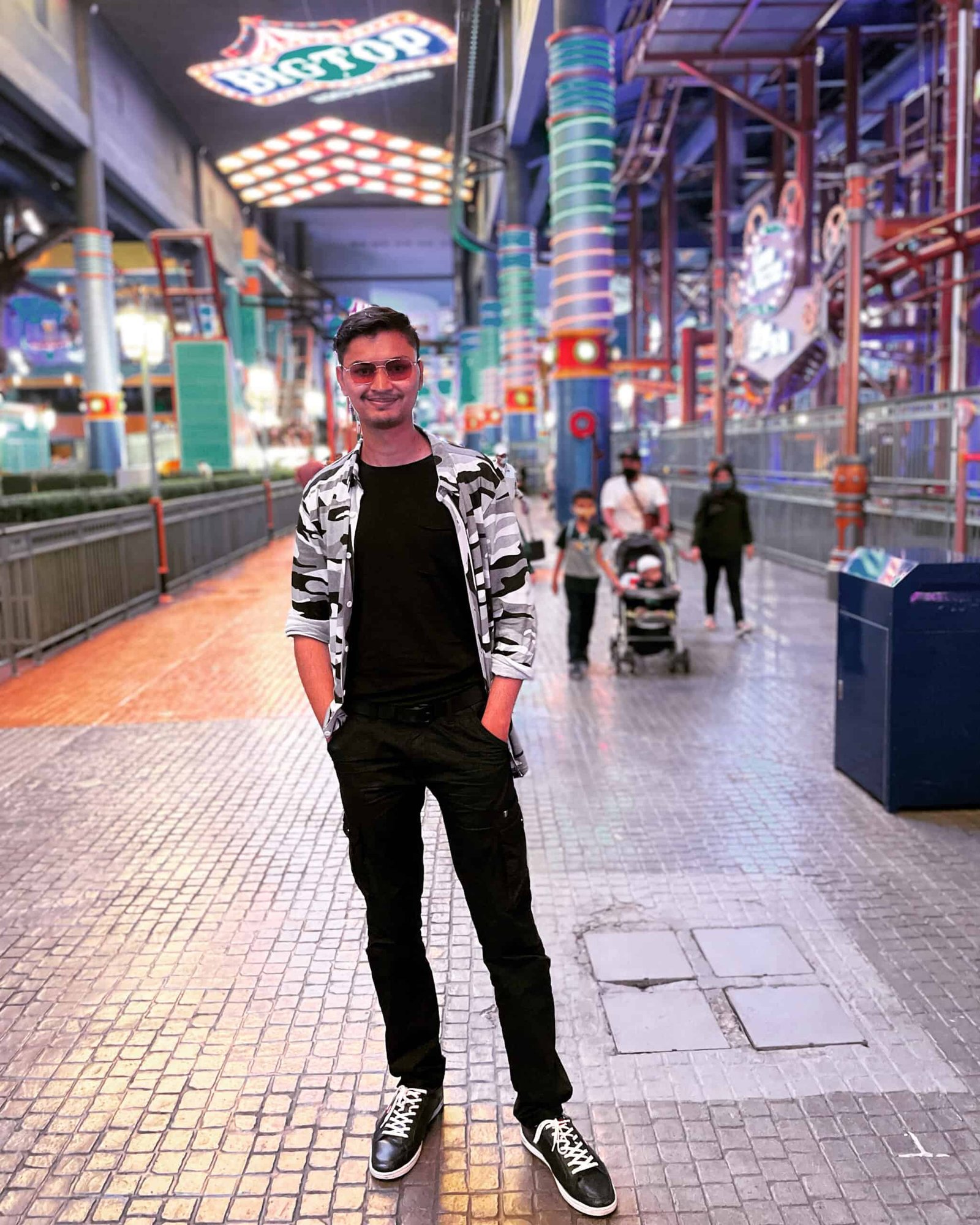
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.