A vector is a data structure that stores multiple values of the same type.
The is.vector() function does not merely check whether an R object is a vector. Instead, it checks whether an input object is a vector with no attributes other than names.
Syntax
is.vector(obj, mode)
Parameters
Name | Value |
obj | It is an input R object that can be a “vector”, “list”, “data frame”, “matrix”, or “array”. |
mode | The mode argument is helpful when you need to differentiate the type of vectors, including numeric, complex, logical, or character vectors. If you don’t specify the mode type, by default, it will check for “any” data type. |
Return value
- It returns TRUE If the input object is a vector with no attributes other than names.
- It returns FALSE If the object has additional attributes (such as dimensions or class) or is not a vector.
Type of modes
Here are the possible values of the “mode” argument:
Atomic modes
- numeric
- logical
- character
- complex
Other modes
- list
- expression
Special modes
- any
Without “mode” argument
Let’s create atomic vectors of different types and check if those are vectors or not.
# Creating a numeric vector
numeric_vector <- c(1, 2, 3)
# Checking if it's a numeric vector
is.vector(numeric_vector) # Output: TRUE
# Creating a character vector
character_vector <- c("apple", "banana", "cherry")
# Checking if it's a character vector
is.vector(character_vector) # Output: TRUE
# Creating a logical vector
logical_vector <- c(TRUE, FALSE)
# Checking if it's a logical vector
is.vector(logical_vector) # Output: TRUE
# Creating a complex vector
complex_vector <- c(1 + 9i, 2 + 1i)
# Checking if it's a complex vector
is.vector(complex_vector) # Output: TRUE
If you apply the is.vector() function to atomic vectors that do not have additional attributes other than names, it will return TRUE.
However, if an atomic vector has additional attributes (For example, “class”), this function will return FALSE.
numeric_vector <- c(1, 2, 3)
attr(numeric_vector, "class") <- "hello"
is.vector(numeric_vector) # Output: FALSE
With “mode” argument
If you create a “logical” vector and pass that vector with mode argument as “logical”, the function will return TRUE. However, if you create a logical vector and pass the mode argument as “numeric” or any type other than “logical”, it will return FALSE.
Ensure that your mode argument aligns with your data type or doesn’t pass at all if not needed.
numeric_vector <- c(11, 21, 3.14, 51)
is.vector(numeric_vector, mode = "numeric") # Output: TRUE
numeric_vector_two <- c(11, 21, 3.14, 51)
is.vector(numeric_vector_two, mode = "complex") # Output: FALSE
logical_vector <- c(TRUE, FALSE, TRUE)
is.vector(logical_vector, mode = "logical") # Output: TRUE
logical_vector_two <- c(TRUE, FALSE, TRUE)
is.vector(logical_vector_two, mode = "numeric") # Output: FALSE
character_vector <- c("apple", "banana", "cherry")
is.vector(character_vector, mode = "character") # Output: TRUE
character_vector_two <- c("apple", "banana", "cherry")
is.vector(character_vector_two, mode = "logical") # Output: FALSE
complex_vector <- c(1 + 2i, 3 - 4i)
is.vector(complex_vector, mode = "complex") # Output: TRUE
complex_vector_two <- c(1 + 2i, 3 - 4i)
is.vector(complex_vector_two, mode = "character") # Output: FALSE
Example of “any” mode
The “any” mode means the function will check a vector of any data type. It doesn’t matter if it’s numeric, character, logical, or complex. As long as it’s a vector, it will return TRUE.
numeric_vector <- c(11, 21, 31)
is.vector(numeric_vector, mode = "any") # Output: TRUE
character_vector <- c("kb", "kl", "yv")
is.vector(character_vector, mode = "any") # Output: TRUE
List and expression
A list is technically a vector because R considers lists recursive vectors, which allow nested elements and are one-dimensional structures that may contain different data types.
main_list <- list(c(1, 2), c("Krunal", "Ankit"))
is.vector(main_list) # TRUE
Let’s evaluate an expression.
expression = "iconic1010"
is.vector(expression) # Output: TRUE
We found practically that R counts the list and expression as a “vector” without attributes other than names.
Data Frames
Data frames are complex data structures consisting of rows and columns.
If you directly check on data frames, is.vector() function returns FALSE. The data frame itself is not a vector.
df <- data.frame(a = c(1, 2), b = c("hello", "world"))
is.vector(df, mode = "any") # Output: FALSE
However, if you check for the data frame’s columns, this function will return TRUE because columns are vectors in the data frame.
df <- data.frame(a = c(1, 2), b = c("hello", "world"))
is.vector(df$a, mode = "any") # Output: TRUE
is.vector(df$b, mode = "any") # Output: TRUE
That’s all!
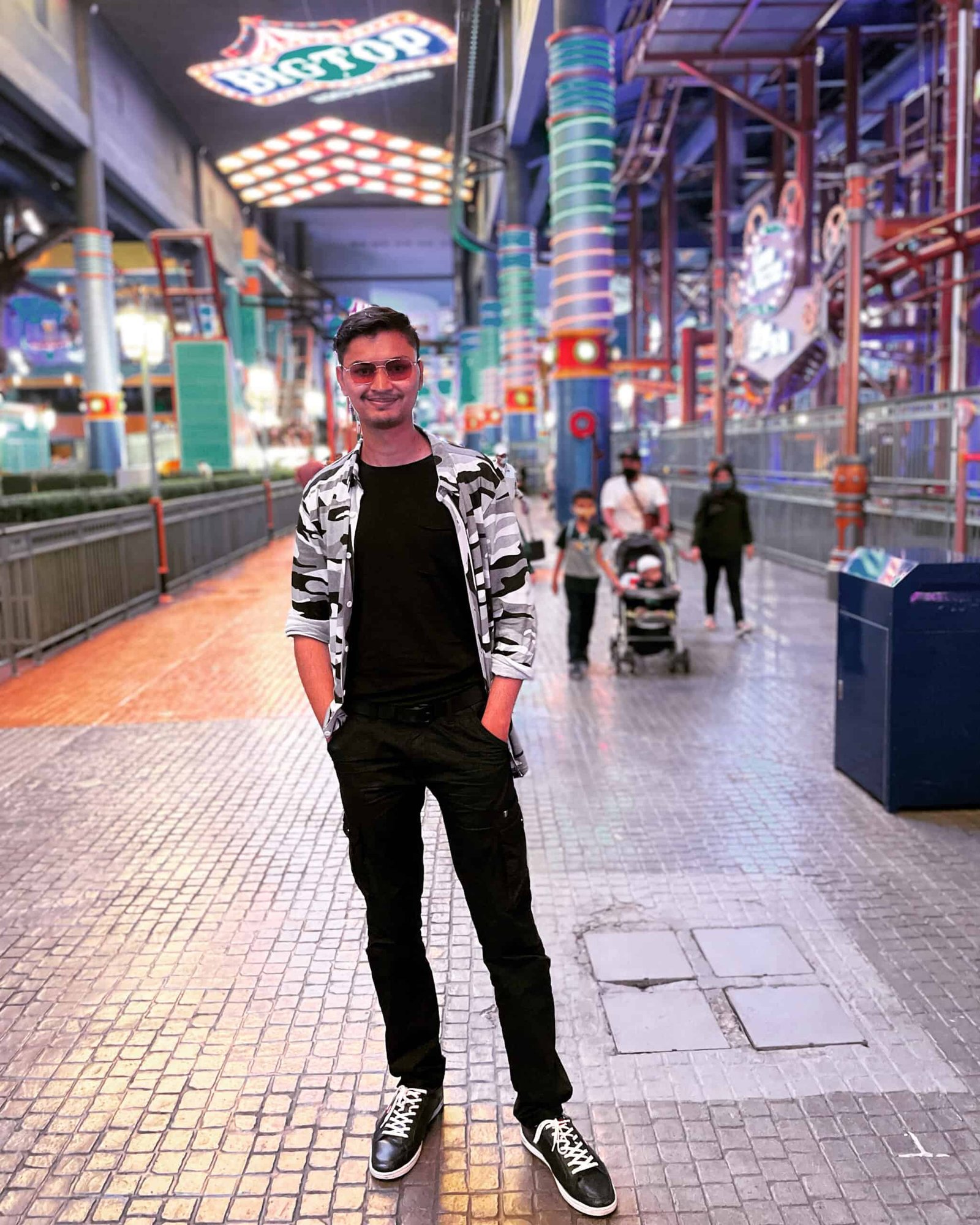
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.