The is.nan() is a built-in R function that checks if a vector or any valid object contains “Not a Number (NaN)” values. It returns a logical vector of the same length as the input, with each element being TRUE if the corresponding element in the input is NaN and FALSE otherwise.
By default, this function works with numeric values.
NaN is a special numeric value in R that results from undefined numerical operations like division by 0 or the square root of a negative value.
Why should we handle NaN values?
Any arithmetic operation involving NaN values results in NaN output because we don’t know what NaN is precisely, which can lead to unexpected errors or incorrect results.
NaN is different from NA and NULL. All these values have a specific purpose.
With the help of is.nan() function, we can identify the source of undefined operations that output NaN while debugging, which helps us prevent unexpected errors.
With this function, you can identify NaN values in your dataset and either remove them or replace them with the mean of that dataset, making your output accurate or near-accurate.
Syntax
is.nan(obj)
Parameters
Name | Value |
obj | It is an input R object that should be a numeric vector by default. |
Numeric Vector
vec <- c(11, NaN, 21, 19, NaN)
print(vec) # [1] 11 NaN 21 19 NaN
is.nan(vec) # [1] FALSE TRUE FALSE FALSE TRUE
You can see in the above code that is.nan() function returns TRUE for NaN values and FALSE otherwise.
If you perform 0 / 0 or any value divided by 0, it will return the NaN value, which you can verify using this function.
zero_div <- 0 / 0
print(vec) # [1] NaN
is.nan(vec) # [1] TRUE
Handling NaN values
Let’s replace NaN values with 0 in the vector (vec) and see the output.
# Replace NaN with 0
vec[is.nan(vec)] <- 0
print(vec) # [1] 11 0 21 19 0
We only assigned 0 to those whose values are NaN.
Lists
The list is a collection of different data types, and when you apply a list as an argument to it.nan() function will give you an error because it cannot handle the list directly.
You must check for each element of the list using the lapply() function, and if that element is NaN, it returns TRUE for that element and FALSE otherwise.
main_list <- list(1, NaN, "KB", c(21, NaN, NaN))
nan_check <- lapply(main_list, \(x) if (is.numeric(x)) is.nan(x) else FALSE)
nan_check
Output
[[1]]
[1] FALSE
[[2]]
[1] TRUE
[[3]]
[1] FALSE
[[4]]
[1] FALSE TRUE TRUE
As you can see:
- The first element of list 19 is not NaN, so it returns FALSE.
- The second element is NaN, so it returns TRUE.
- The third element is “KB”, which is not even a numeric value, so it returns FALSE.
- The fourth element is a numeric vector containing other numeric values. So, it will check for each element, and if NaN is found, it returns TRUE; otherwise, it returns FALSE. If the last vector contained character values, it would have returned FALSE.
Matrix
This function checks element-wise in the matrix like the vector and returns TRUE for NaN and FALSE otherwise.
main_mtrx <- matrix(c(11, 19, NaN, 21), nrow = 2)
main_mtrx
is.nan(main_mtrx)
Output
Data Frame
Data Frame is a complex data type you cannot use directly.nan() function with it. What you can do is pass an individual column of a data frame, and then it will act as a vector, and this function will check if it contains NaN.
df <- data.frame(a = c(1, NaN, 3), b = c(4, 5, NaN), c = c("a", "b", NaN))
# is.nan(df)
# Output: Error in is.nan(df) : default method not implemented for type 'list'
# Correct way: apply to columns
is.nan(df$a)
# Output: [1] FALSE TRUE FALSE
is.nan(df$b)
# Output: [1] FALSE FALSE TRUE
is.nan(df$c)
# Output: [1] FALSE FALSE FALSE
In the above code, we directly applied our function to df, which resulted in an error. The code is already commented.
Then, we started applying a function to individual columns, and that’s where it is working correctly, and we get the output vector of the same size as the column’s size.
That’s all, mates!
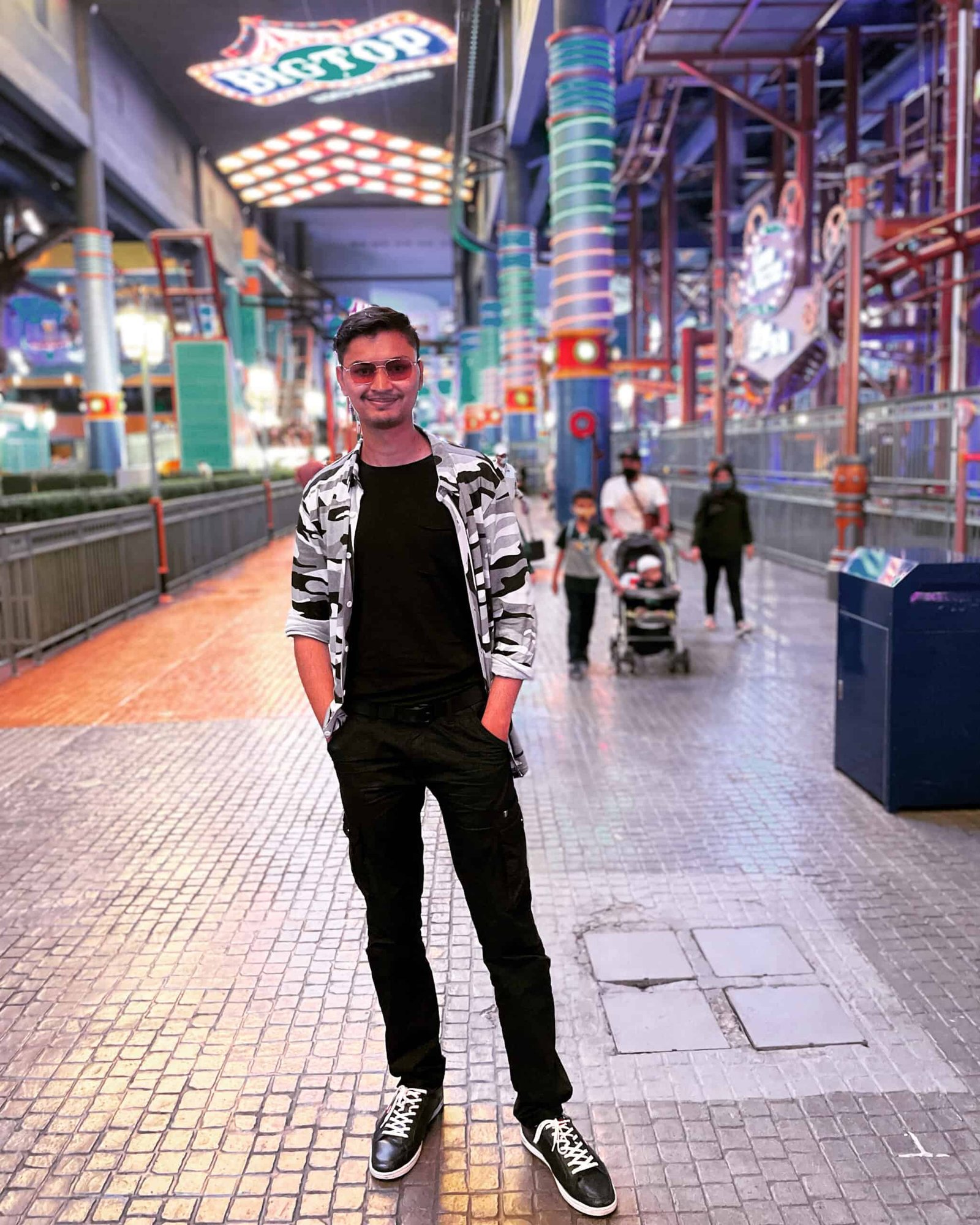
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.