If you want to put data validation in progress, you must identify the file type or “MIME Type” before processing. To identify the file type, we might need to check its extension.
Here are two ways to get an extension of a file in R:
- Using the tools::file_ext()
- Using regular expression
Method 1: Using the tools::file_ext()
The file_ext() is not a base R’s but a “tools” package’s method. It accepts “file path” and returns only the extension of the file.
We must install the package if we have to use it.
install.packages("tools")
Load the package like this:
library("tools")
Visual representation
Before getting the extension of a file, you need to check if a file exists.
Use the file.exists() method to check for a file’s existence. If it exists, you can use the file_ext() function.
Example
library(tools)
file <- "dataframe.R"
if (file.exists(file)) {
file_ext(file)
} else {
cat("The file does not exist")
}
Output
[1] "R"
Method 2: Using regular expression
If you are not keen on using third-party packages, then old ways to use “Regular Expressions”. R provides a sub() or gsub() method to create a regex pattern to help us extract extension from the file name.
Example
file <- "dataframe.R"
extension <- sub(".*\\.", "", file)
print(extension)
Output
[1] "R"
Getting a filename without an extension
If you have a requirement to extract a filename without extension, you can use the “file_path_sans_ext()” method. Unfortunately, the file_path_sans_ext() method is not a built-in method, and you also have to install and import the “tools” package first.
Example
library(tools)
file <- "dataframe.R"
if (file.exists(file)) {
file_path_sans_ext(file)
} else {
cat("The file does not exist")
}
Output
[1] "dataframe"
You can also use the basename() function to remove the path leading to the file, and with this regex, any extension will be removed.
library(tools)
file <- "dataframe.R"
if (file.exists(file)) {
sub(pattern = "(.*)\\..*$", replacement = "\\1", basename(file))
} else {
cat("The file does not exist")
}
Output
[1] "dataframe"
That is it.
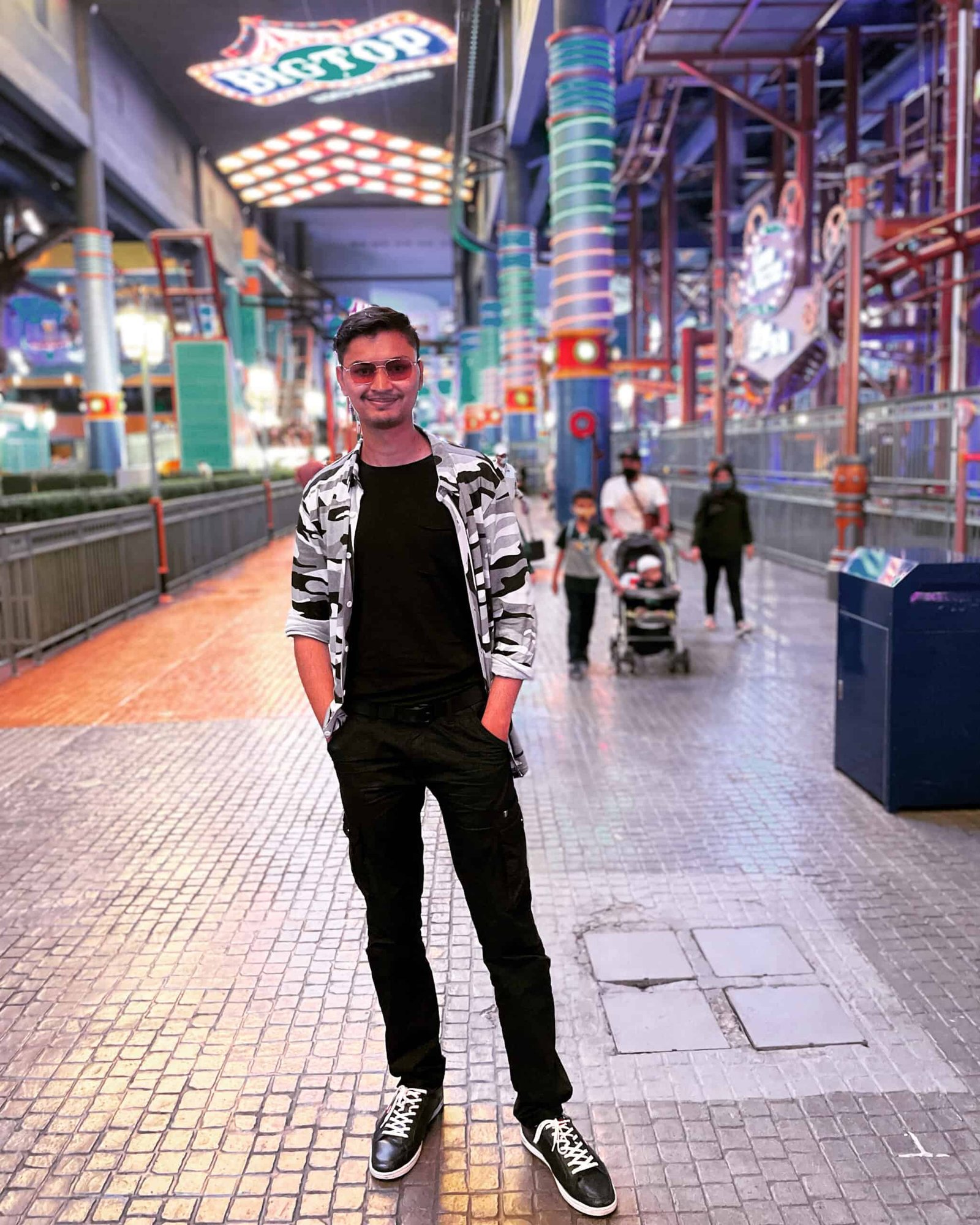
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.