Whether you are reading or writing files via programs in the file system, it is necessary to check if that file already exists. If you don’t put a check in place, it can lead to either a program crash or an error.
Checking if the file exists
The most efficient way to check if the file exists is to use the built-in file.exists() method. It accepts a path that can lead to a file or directory and returns TRUE if it is there and FALSE otherwise. You can see all of these in the above figure.
Assume that we have a directory called “newDir”, and in that directory, we have a file called “test.R”.
Let’s check programmatically if the test.R file is there in the newDir directory:
file <- "newDir/test.R"
if (file.exists(file)) {
cat("The file is there", "\n")
} else {
cat("The file does not exist", "\n")
}
# Output: The file is there
Since the file already exists, as we can see from the above screenshot, the if condition executes.
Let’s consider another scenario where we check for the file name new.R, which we know doesn’t exist inside the newDir folder.
file <- "newDir/new.R"
if (file.exists(file)) {
cat("The file is there", "\n")
} else {
cat("The file does not exist", "\n")
}
# Output: The file does not exist
By default, the method searches in the current working directory of RStudio that is currently in use.
To search in other directories, you must provide the complete file path.
Checking multiple files’ existence
The file.exists() function is vectorized, meaning you can pass multiple files at once as a vector, and it will check the existence for each one and return TRUE if it exists and FALSE otherwise.
The output will be a logical vector containing TRUE or FALSE values, and it has the same length as the input.
Let’s assume that in our newDir directory, we removed the test.R file and created three new files and one folder:
The above screenshot of the newDir directory shows one folder and three files: one.txt, two.txt, and three.txt.
We will check the existence of these files (which we know exist) non-existent files:
file1 <- "newDir/one.txt"
file2 <- "newDir/five.txt"
file3 <- "newDir/four.txt"
file4 <- "newDir/two.txt"
file5 <- "newDir/three.txt"
output_vec <- file.exists(c(file1, file2, file3, file4, file5))
output_vec
# Output: [1] TRUE FALSE FALSE TRUE TRUE
From the above output, you can see that only the first, fourth, and fifth files exist, and it returns TRUE for that. For non-existent, it returns FALSE.
Symbolic links
The file.exists() function supports symbolic links, and it can resolve it and check for the target’s existence.
file.exists(Sys.readlink("symlink_to_file"))
Checking file readability
If the file exists, you can read it using the read.delim() function.
if (file.exists("newDir/test.R") == 0) {
data <- read.delim("newDir/test.R")
}
fs package
There is a specific file system package called “fs” that you can use to manage file system tasks. For example, it provides the file_exists() function to check if the file exists.
library(fs)
file1 <- "newDir/one.txt"
file2 <- "newDir/five.txt"
file3 <- "newDir/four.txt"
file4 <- "newDir/two.txt"
file5 <- "newDir/three.txt"
fs::file_exists(c(file1, file2, file3, file4, file5))
# Output:
# newDir/one.txt newDir/five.txt newDir/four.txt newDir/two.txt newDir/three.txt
# TRUE FALSE FALSE TRUE TRUE
file.test()
The file_test() function performs shell-style file tests. It can be used to test for the existence of a file, its type, and the permissions on a file.
library(utils)
file <- "/Users/krunallathiya/Desktop/Code/pythonenv/env/newDir/test.R"
file_test("-f", file)
# Output: [1] TRUE
It returns TRUE because the file is inside the newDir directory.
Let’s check for another file called new.R inside the newDir directory.
Using the file_test() function, we can check if a new.R file exists inside the newDir directory.
library(utils)
file <- "/Users/krunallathiya/Desktop/Code/pythonenv/env/newDir/new.R"
file_test("-f", file)
# Output: [1] FALSE
FALSE means that the file does not exist.
Checking if the folder exists
There is a built-in specific function called “dir.exists()” that you can use to check if the directory already exists. It returns only TRUE if the directory exists and FALSE otherwise.
In our “newDir” folder, we have a “folder” directory.
Let’s check for that:
dir.exists("newDir/folder")
# Output: [1] TRUE
And it returns TRUE. If we check for the non-existent directory, it returns FALSE.
dir.exists("newDir/docs")
# Output: [1] FALSE
file.exists()
The file.exists() function not only checks for files but also checks for directories too. It returns TRUE for existence and FALSE otherwise.
file.exists("newDir/folder")
# Output: [1] TRUE
Multiple directories checking
For checking multiple directories, pass a vector of directories to the file.exists() function.
dir1 <- "newDir/folder"
dir2 <- "newDir/folder2"
dir3 <- "newDir/folder3"
file.exists(dir1, dir2, dir3)
# Output: [1] TRUE FALSE FALSE
That’s all!
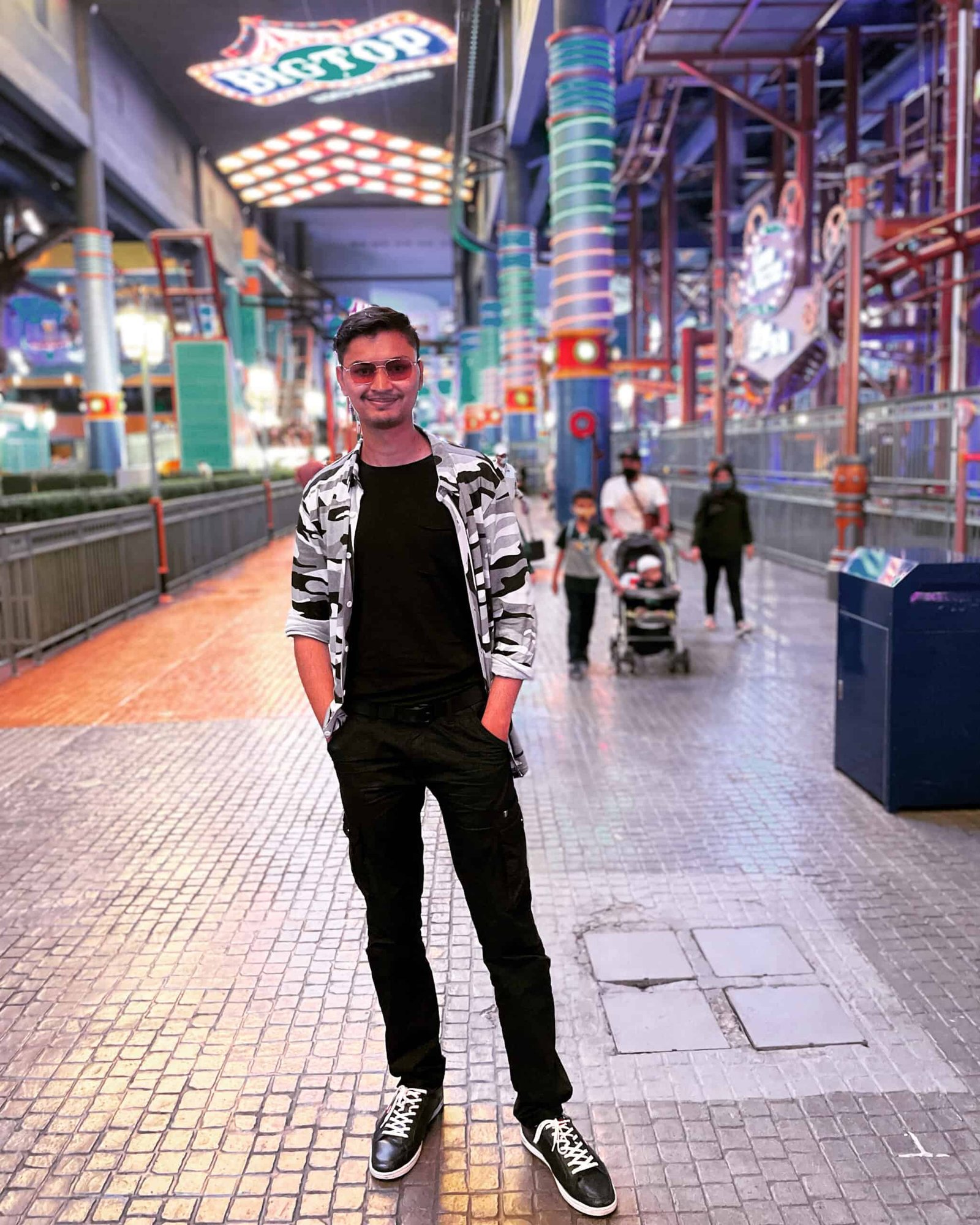
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.