The most efficient and idiomatic way to check if a vector is empty in R is by using the built-in length() function and checking whether its length is 0. If “length(vec) == 0” returns TRUE, the vector is empty; otherwise, it is not empty.
An empty vector means it has no elements. One thing to note is that even if a vector is empty, it can still have a data type. For example, you can create an empty numeric vector using the numeric() function or the character() function to create a character vector.
# Defining an empty vector
empty_vector <- c()
# Checking if the vector is empty
is_empty <- length(empty_vector) == 0
# Printing the result
print(is_empty)
# Output
# TRUE
Since the vector is empty, we got TRUE as output.
Let’s check out for a non-empty vector:
# Defining a non-empty vector
non_empty_vector <- c(1, 2, 3)
# Checking if the vector is empty
is_empty <- length(non_empty_vector) == 0
# Printing the result
print(is_empty)
# Output
# FALSE
Checking with a conditional statement
The length(vec) == 0 returns a boolean result, which is why we can use this type of code snippet in the if-else statement to process further operations.
# Defining a non-empty vector
non_empty_vector <- c("KB", "KL")
# Checking if the vector is empty using a conditional statement
if (length(non_empty_vector) == 0) {
print("The vector is empty!")
} else {
print("The vector is not empty")
}
Output
The vector is not empty
If you check for an empty vector, it will return the below output.
[1] The vector is empty!
Why should we check?
If you want to write robust, reliable, and error-free code that will prevent any future unexpected errors, you should always check whether your input object or vector is empty. It is considered best practice to include all types of unforeseen scenarios.
Difference between NULL vector and empty vector
An empty vector is not the same as a NULL vector.
The main difference is that the NULL vector itself represents the absence of an object, whereas an empty vector just does not contain any element, but it is still an R object.
# Defining empty vector
empty_vec <- c()
is.null(empty_vec)
# Output: FALSE
# Defining NULL object
null_object <- NULL
is.null(null_object)
# Output: TRUE
The is.null() function returns TRUE for a NULL object, not an empty object.
Shorter way to check
You can also use the shorter syntax “!length(vec)”.
# Defining empty vector
empty_vec <- c()
# Checking if a Vector is empty using shorter syntax
if (!length(empty_vec)) {
print("It is empty")
} else {
print("It is not empty")
}
# Defining a non-empty vector
non_empty_vector <- c(19, 21)
# Checking if It is empty using shorter syntax
if (!length(non_empty_vector)) {
print("It is empty")
} else {
print("It is not empty")
}
Output
[1] "It is empty"
[1] "It is not empty"
That’s all
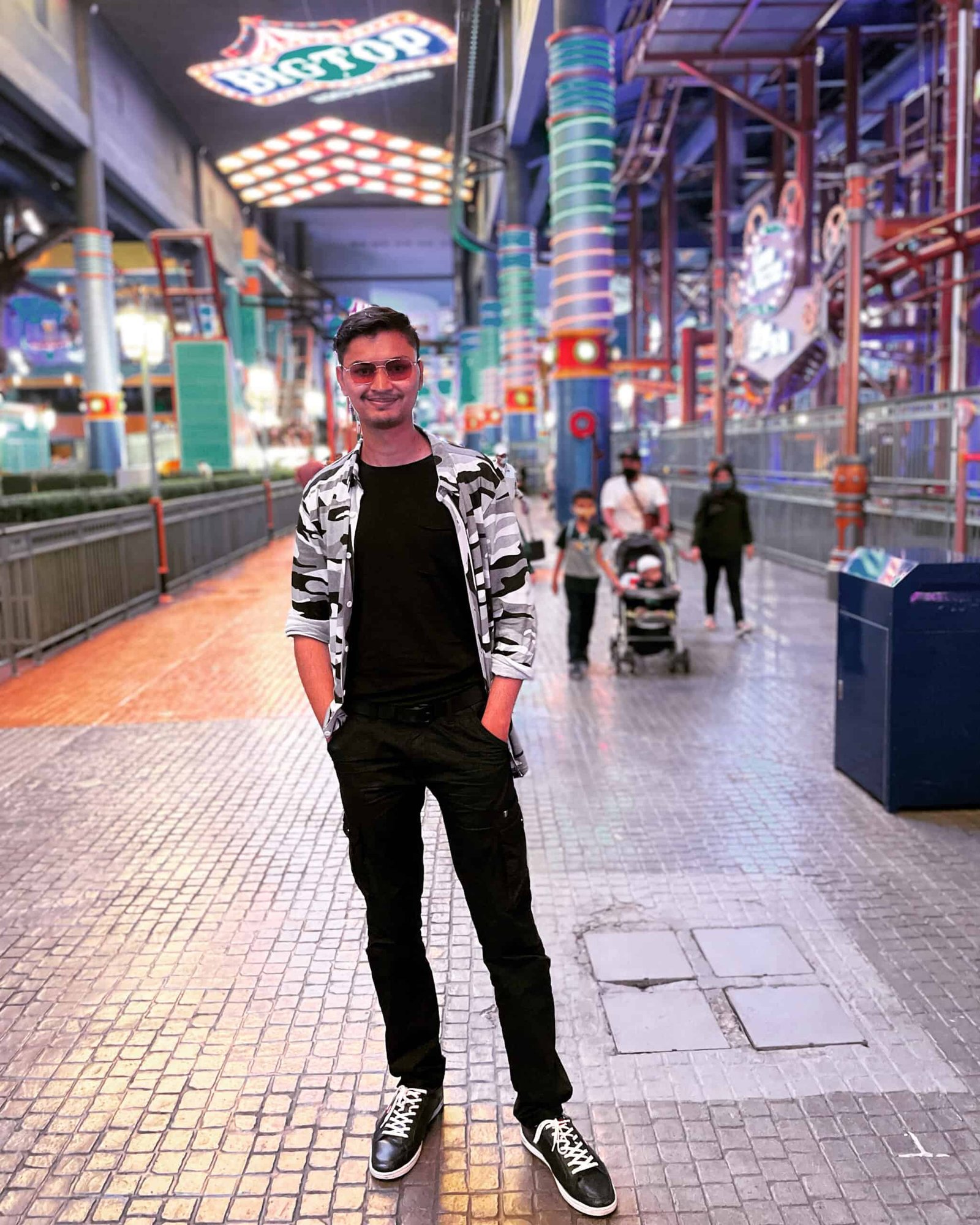
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.