The as.numeric() function in R converts valid non-numeric data into numeric data.
What do I mean by valid non-numeric data? They are valid numeric values that are stored as text or categorical variables (factors). For example, “1” is a valid non-numeric data but “A” is not valid non-numeric data as far as the as.numeric() method is concerned.
If the character values are literal characters, this method won’t be able to convert them to numeric. If you use it on non-numeric characters, it returns NA for those values.
The primary purpose of this method is to convert a suitable factor, vector, character (posed as numericals), or logical to numeric values.
Syntax
as.numeric(character)
Parameters
Argument | Description |
character | It can be a character, a logical factor, or a list. |
Character vector to numeric
Let’s define a vector containing characters, but those characters are underlying numeric values.
If R can correctly interpret character values as numeric, it will convert successfully.
vec <- c("1", "2", "3")
as.numeric(vec)
# Output: [1] 1 2 3
You can use the is.numeric() method to check if the value is numeric.
vec <- c("1", "2", "3")
numeric_vec <- as.numeric(vec)
is.numeric(numeric_vec)
# Output: [1] TRUE
Factor
As we already know, factors are categorical variables represented as integers with corresponding labels.
Here, we are more focused on the underlying factor levels, which are numeric values.
You can see from the above figure that even if the labels are character vectors, when you pass the factor to the as.numeric() method, it will return factor levels of the factor, which are the numeric values.
In the factor type, each level, represented by a unique string value, is replaced by its corresponding integer code. Factor levels are encoded as integers starting with 1.
factor_var <- factor(c("A", "B", "C"))
numeric_var <- as.numeric(factor_var)
print(numeric_var)
# Output: [1] 1 2 3
Logical vector
The logical vector can only contain two values: True and False.
If you pass this vector to the as.numeric() method, True becomes 1 and False becomes 0, which is a logical way to look at it.
vec_logical <- c(FALSE, FALSE, TRUE, TRUE)
vec_num <- as.numeric(vec_logical)
vec_num
# Output: [1] 0 0 1 1
List
What if your input is a list? Well, that list should contain valid values to convert to a number.
In this figure, we obtain the numeric vector directly in the output without needing the unlist() function.
num_list <- list(11, 21, 19, 46)
num_vec <- as.numeric(num_list)
num_vec
# Output: [1] 11 21 19 46
If our input list contains character values, it will return a vector filled with NAs; otherwise, it will return a vector filled with proper numeric values.
The above figure illustrates that when passing a list of mixed values, including both numeric and character values, character values are converted to NA, while numeric values remain unchanged.
num_list <- list(11, "KL", 19, "KB")
number <- as.numeric(num_list)
number
# Output:
# Warning messages:
# 1: NAs introduced by coercion
# 2: NAs introduced by coercion
# [1] 11 NA 19 NA
Plotting
Let’s plot a chart of the town’s temperature based on the date (day by day).
To plot a chart, we need to convert character dates to Date objects and then convert the dates into numeric values using the as.numeric() method.
dates <- c("2024-09-21", "2024-09-22", "2024-09-23", "2024-09-24", "2024-09-25")
temperatures <- c(5, 8, 12, 15, 20)
# Converting character dates to Date objects
date_objects <- as.Date(dates)
# Converting dates to numeric (days since 1970-01-01)
date_numeric <- as.numeric(date_objects)
# Plotting
plot(date_numeric, temperatures, type = "b", xaxt = "n",
main = "Temperature Over Time")
axis(1, at = date_numeric, labels = format(date_objects, "%b %d"), las = 2)
That’s it!
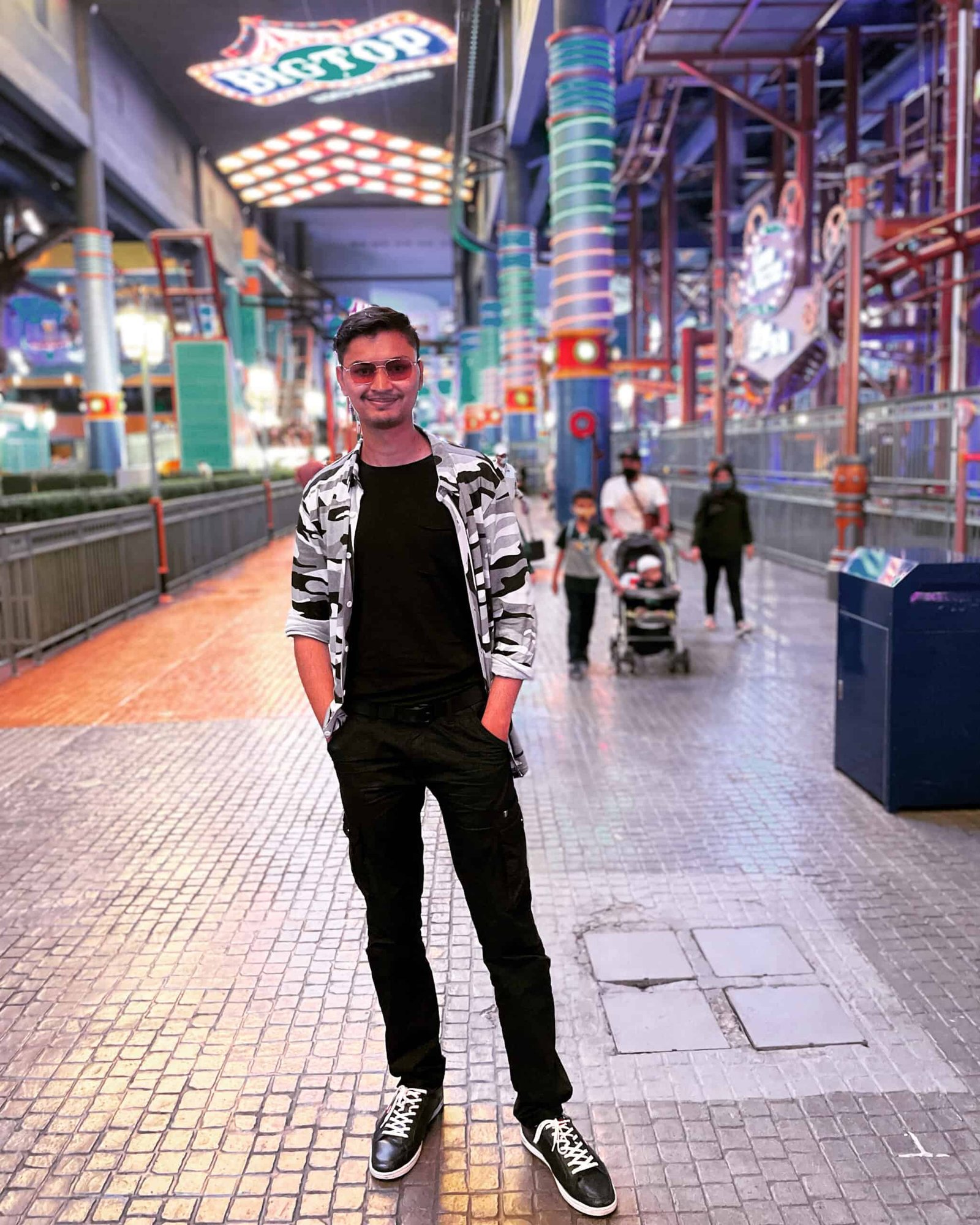
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.