When you convert a list to a matrix, you are essentially taking the elements of a list and rearranging them into a two-dimensional structure with rows and columns.
To convert a list to the matrix in R, use the matrix() function and pass the unlist() function as an argument. The unlist() function flattens a list into a single vector, and then the matrix() function accepts this vector and reshapes it into a matrix with the specified number of rows and columns.
Syntax
# convert list to matrix (by row)
matrix(unlist(my_list), ncol=3, byrow=TRUE)
# convert list to matrix (by column)
matrix(unlist(my_list), ncol=3)
List with Equal Lengths and Same Data Types
Converting by columns
By default, if all elements of the list have the same length and you don’t pass any argument, you will get a matrix by column.
main_list <- list(1:3, 4:6, 7:9)
mtrx <- matrix(unlist(main_list), ncol = 3)
print(mtrx)
Output
[,1] [,2] [,3]
[1,] 1 4 7
[2,] 2 5 8
[3,] 3 6 9
Converting by rows
To convert a list to a matrix by rows, pass the byrow = TRUE to the matrix() function.
main_list <- list(1:3, 4:6, 7:9)
mtrx <- matrix(unlist(main_list), ncol = 3, byrow = TRUE)
print(mtrx)
Output
[,1] [,2] [,3]
[1,] 1 2 3
[2,] 4 5 6
[3,] 7 8 9
List with equal lengths but different data types
If you have a list that has elements of the same length but different data types, R will coerce the elements to a common type, usually character.
main_list <- list(c(1, 2, 3), c("a", "b", "c"), c(TRUE, FALSE, TRUE))
converted_matrix <- matrix(unlist(main_list), nrow = 3, byrow = TRUE)
print(converted_matrix)
Output
List with elements of unequal lengths
Let’s create a list of unequal lengths and pass that to the matrix() function.
main_list <- list(1:4, 6:9, 10:12)
matrix(unlist(main_list), ncol = 3)
Output
[,1] [,2] [,3]
[1,] 1 6 10
[2,] 2 7 11
[3,] 3 8 12
[4,] 4 9 1
Warning message:
In matrix(unlist(main_list), ncol = 3) :
data length [11] is not a sub-multiple or multiple of the number of rows [4]
Here, you can see that we got the warning message: In matrix(unlist(main_list), ncol = 3): data length [11] is not a sub-multiple or multiple of the number of rows [4].
It simply means that when we convert it into a vector and then convert it into a matrix, it does not have enough elements to create a matrix, so by default, it repeats the element to complete the matrix.
Bonus: Using do.call() and rbind() or cbind()
If your list contains elements (like vectors) that you want to be rows or columns of the matrix, you can use do.call() with rbind() or cbind().
# Assuming your list
main_list <- list(1:4, 6:9, 10:13)
# Convert list to matrix (each list element becomes a row)
main_matrix <- do.call(rbind, main_list)
main_matrix
Output
[,1] [,2] [,3]
[1,] 1 6 10
[2,] 2 7 11
[3,] 3 8 12
[4,] 4 9 13
That’s it.
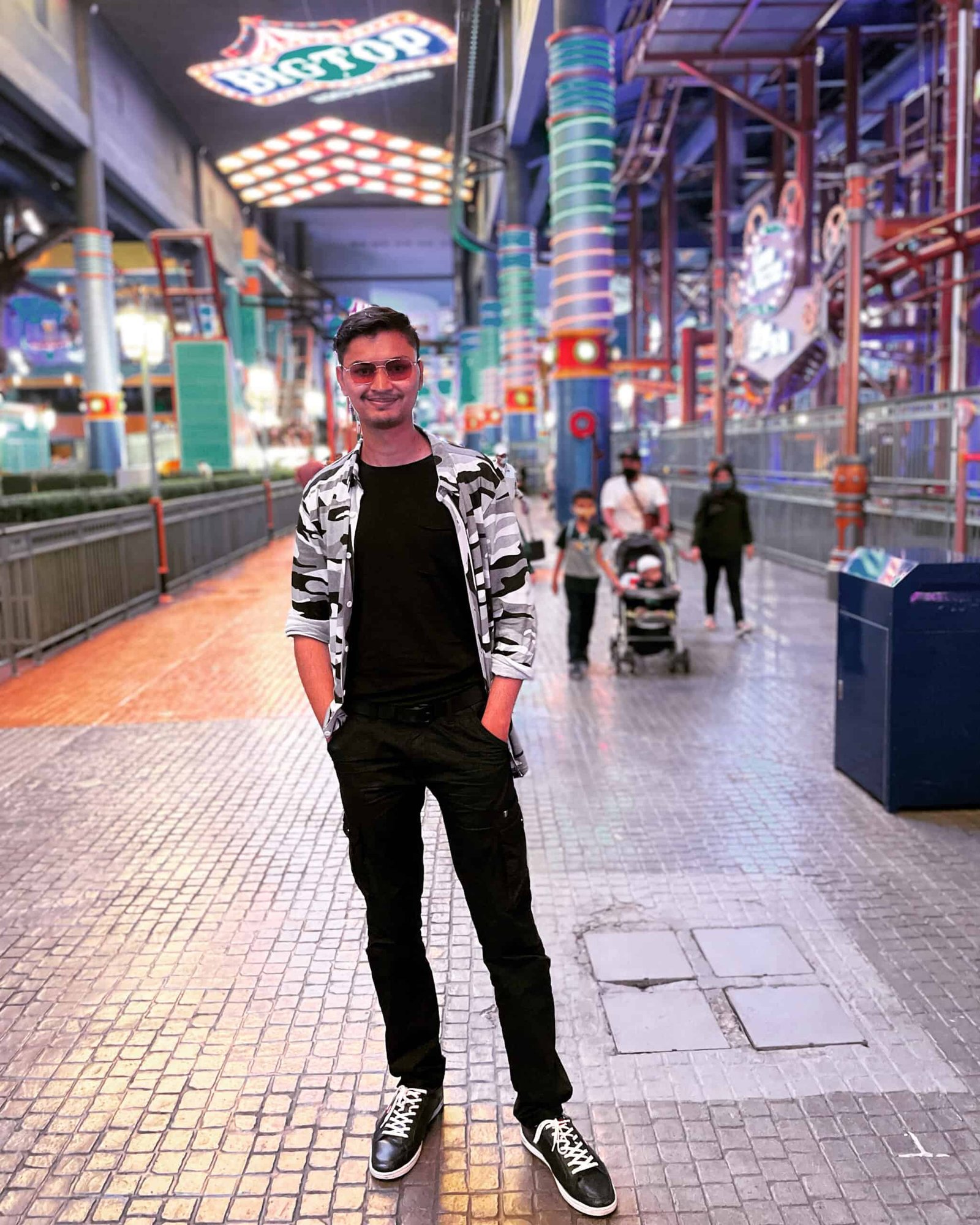
Krunal Lathiya is a seasoned Computer Science expert with over eight years in the tech industry. He boasts deep knowledge in Data Science and Machine Learning. Versed in Python, JavaScript, PHP, R, and Golang. Skilled in frameworks like Angular and React and platforms such as Node.js. His expertise spans both front-end and back-end development. His proficiency in the Python language stands as a testament to his versatility and commitment to the craft.